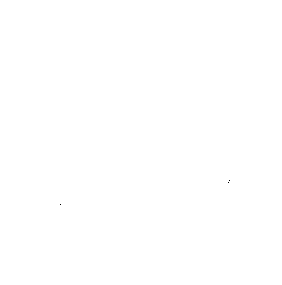
Chakram is a REST API testing framework
offering a BDD testing style and fully exploiting promises
offering a BDD testing style and fully exploiting promises
Simple API Testing
Chakram allows you to write clear and comprehensive tests,
ensuring JSON REST endpoints work correctly as you develop and in the future
HTTP Assertions
Chakram extends Chai.js, adding HTTP specific assertions. It allows simple verification of returned status codes, the compression used, cookies, headers, returned JSON objects and the schema of the JSON response (using the JSON schema specification).describe("HTTP assertions", function () {
it("should make HTTP assertions easy", function () {
var response = chakram.get("http://httpbin.org/get?test=chakram");
expect(response).to.have.status(200);
expect(response).to.have.header("content-type", "application/json");
expect(response).not.to.be.encoded.with.gzip;
expect(response).to.comprise.of.json({
args: { test: "chakram" }
});
return chakram.wait();
});
});
Promises
API testing is naturally asynchronous, which can make tests complex and unwieldy. Chakram fully exploits javascript promises, resulting in clear asynchronous tests.describe("Promises", function () {
it("should support asserting Biggie's best track", function () {
var artist = "Notorious B.I.G.";
return chakram.get("https://api.spotify.com/v1/search?q="+artist+"&type=artist")
.then(function (searchResponse) {
var bigID = searchResponse.body.artists.items[0].id;
return chakram.get("https://api.spotify.com/v1/artists/"+bigID+"/top-tracks?country=GB");
})
.then(function (topTrackResponse) {
var topTrack = topTrackResponse.body.tracks[0];
expect(topTrack.name).to.contain("Old Thing Back");
});
});
});
BDD + Hooks
Using BDD formatting and hooks, complex tests can be constructed with the necessary setup and tear down operations, all described in natural language for improved clarity and maintenance.describe("BDD + Hooks", function () {
var thingName;
before("post dweet", function () {
thingName = "chakramtest" + Math.floor(Math.random()*2000);
return chakram.post("https://dweet.io/dweet/for/"+thingName, {
testing: "your API"
});
});
it("should support getting latest dweet", function () {
var postedData = chakram.get("https://dweet.io/get/latest/dweet/for/"+thingName);
return expect(postedData).to.have.json('with[0].content', {
testing: "your API"
});
});
after("update dweet with result", function () {
return chakram.post("https://dweet.io/dweet/for/"+thingName, {
testing: "passed"
});
});
});
Extensibility
Chakram allows new assertions to be added to the framework. This is ideal for adding edge case HTTP assertions or project specific assertions. The end result is less duplication and clearer tests.describe("Extensibility", function () {
before("define teapot", function () {
chakram.addProperty("teapot", function (respObj) {
var statusCode = respObj.response.statusCode;
this.assert(statusCode === 418,
'expected status code ' + statusCode + ' to equal 418',
'expected ' + statusCode + ' to not be equal to 418');
});
});
it("should be able to detect teapots", function () {
var notATeapot = chakram.get("http://httpbin.org/status/200");
var aTeapot = chakram.get("http://httpbin.org/status/418");
expect(notATeapot).to.not.be.teapot;
expect(aTeapot).to.be.teapot;
return chakram.wait();
});
});
Getting Started
Chakram runs on node.js and is distributed via NPM. To begin testing, a node.js application should be created to contain the API tests and Chakram added as a development dependency:npm install --save-dev chakram
The Mocha test runner is used to run Chakram tests – this can be installed globally or as a development dependency. The following command installs Mocha globally:
npm install -g mocha
To run tests, simply call the Mocha command line tool. By default, this will run the tests located in the 'test' directory. Mocha can export the test results in many different formats, satisfying the majority of continuous integration platforms.
Examples, tests and documentation are available to introduce Chakram's capabilities.
Issues, pull requests and questions are welcomed – happy testing!